Apollo vs Urql vs Fetch: The Ultimate Showdown
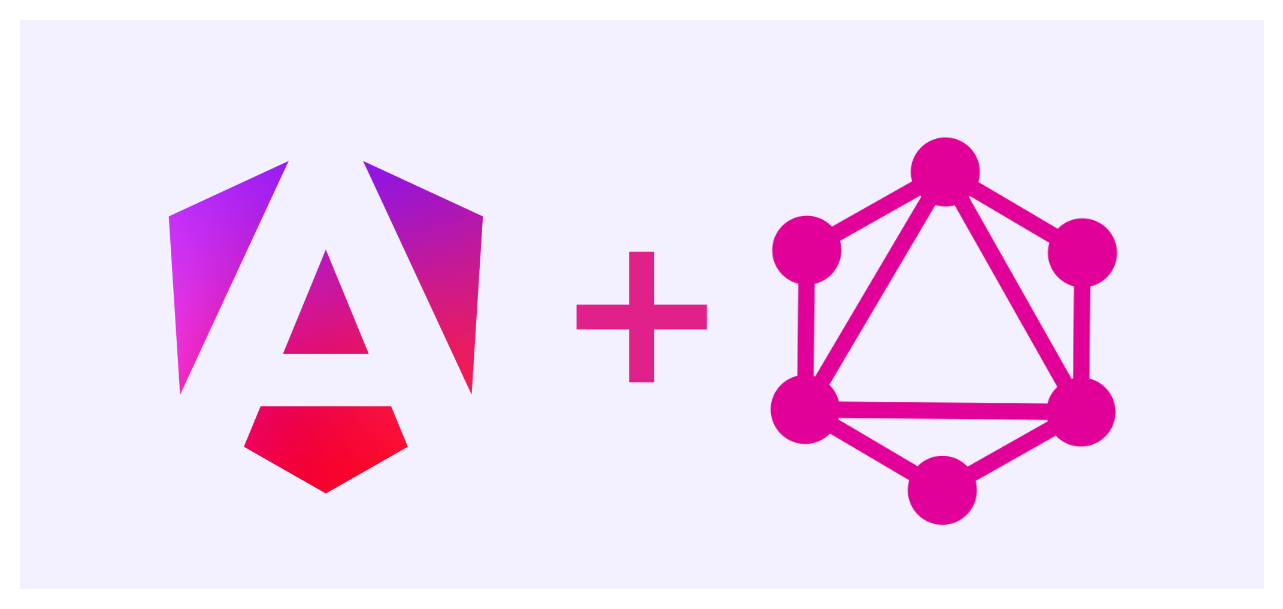
Angular developers often face the challenge of efficiently fetching and managing data from GraphQL APIs. This comprehensive guide dives into five powerful approaches for integrating GraphQL into your Angular applications. We'll explore everything from full-featured client libraries to lightweight solutions, using a practical example of fetching post data to demonstrate each method's strengths and nuances.
Our journey will take us through Apollo Angular, Urql, GraphQL-Request, Axios, and the native Fetch API, each offering unique advantages for different project needs. Whether you're building a small-scale application or a complex enterprise system, this guide will equip you with the knowledge to choose the best GraphQL integration method for your Angular project.
We'll not only cover the implementation details but also delve into error handling strategies, providing you with robust solutions to gracefully manage various API-related issues. By the end of this guide, you'll have a clear understanding of how to leverage GraphQL in Angular, complete with code snippets, real-world analogies, and a detailed comparison table to aid your decision-making process.
So, buckle up and get ready to supercharge your Angular applications with the power of GraphQL!
NB: We are not using the traditional NgModule-based Angular applications instead we will be using the newer standalone component approach; below is the version of angular cli version used throughout the guide.
ng version
_ _ ____ _ ___
/ \ _ __ __ _ _ _| | __ _ _ __ / ___| | |_ _|
/ △ \ | '_ \ / _` | | | | |/ _` | '__| | | | | | |
/ ___ \| | | | (_| | |_| | | (_| | | | |___| |___ | |
/_/ \_\_| |_|\__, |\__,_|_|\__,_|_| \____|_____|___|
Angular CLI: 18.0.7
Node: 20.12.2
Package Manager: npm 10.5.0
OS: linux x64
Angular: 18.0.6
... animations, common, compiler, compiler-cli, core, forms
... platform-browser, platform-browser-dynamic, platform-server
... router
Package Version
---------------------------------------------------------
@angular-devkit/architect 0.1800.7
@angular-devkit/build-angular 18.0.7
@angular-devkit/core 18.0.7
@angular-devkit/schematics 18.0.7
@angular/cli 18.0.7
@angular/ssr 18.0.7
@schematics/angular 18.0.7
rxjs 7.8.1
typescript 5.4.5
zone.js 0.14.7
We'll be using a Tailcall backend that wraps the JSONPlaceholder API, providing a GraphQL interface to RESTful data.
🛠️ Project Setup
First, let's set up our Angular project:
ng new angular-graphql-tailcall-showcase
cd angular-graphql-tailcall-showcase
🔧 Tailcall Backend Configuration
Create a tailcall
directory in the project root and add a jsonplaceholder.graphql file:
# File: tailcall/jsonplaceholder.graphql
schema
@server(port: 8000, hostname: "0.0.0.0")
@upstream(httpCache: 42) {
query: Query
}
type Query {
posts: [Post]
@http(url: "http://jsonplaceholder.typicode.com/posts")
user(id: Int!): User
@http(
url: "http://jsonplaceholder.typicode.com/users/{{.args.id}}"
)
}
type User {
id: Int!
name: String!
username: String!
email: String!
phone: String
website: String
}
type Post {
id: Int!
userId: Int!
title: String!
body: String!
user: User
@http(
url: "http://jsonplaceholder.typicode.com/users/{{.value.userId}}"
)
}
To start the Tailcall server, run:
tailcall start ./tailcall/jsonplaceholder.graphql
1. Apollo Angular - The Luxury Sports Car of GraphQL Clients
First up on our list is Apollo Angular. If GraphQL clients were cars, Apollo would be the Tesla of the bunch - sleek, powerful, and packed with features you didn't even know you needed. Let's pop the hood and see what makes this beauty purr!
Installation and Integration Steps
Before we can take Apollo for a spin, we need to get it set up in our garage (I mean, project). Here's how:
-
Install the necessary packages::
npm install apollo-angular @apollo/client graphql
-
Configure Apollo in your
app.config.ts
:import { APOLLO_OPTIONS, ApolloModule } from 'apollo-angular';
import { HttpLink } from 'apollo-angular/http';
import { InMemoryCache } from '@apollo/client/core';
// In your ApplicationConfig
{
providers: [
importProvidersFrom(ApolloModule),
{
provide: APOLLO_OPTIONS,
useFactory: (httpLink: HttpLink) => ({
cache: new InMemoryCache(),
link: httpLink.create({
uri: '/graphql',
}),
}),
deps: [HttpLink],
},
],
} -
Code Snippets Now that we've got our Apollo rocket fueled up, let's see it in action! Here's a component that fetches a list of posts using Apollo in
src/app/apollo-angular/post-list.component.ts
:
import {Component, OnDestroy} from "@angular/core"
import {CommonModule} from "@angular/common"
import {Apollo, gql} from "apollo-angular"
import {ChangeDetectorRef} from "@angular/core"
import {
catchError,
takeUntil,
mergeMap,
} from "rxjs/operators"
import {Subject, of, throwError} from "rxjs"
@Component({
selector: "app-apollo-post-list",
standalone: true,
imports: [CommonModule],
template: `
<h2>Posts (Apollo Angular)</h2>
<button (click)="fetchPosts()" [disabled]="loading">
{{ loading ? "Loading..." : "Load Posts" }}
</button>
<button (click)="triggerNetworkError()">
Trigger Network Error
</button>
<button (click)="triggerGraphQLError()">
Trigger GraphQL Error
</button>
<button (click)="triggerUnexpectedError()">
Trigger Unexpected Error
</button>
<ul *ngIf="!error">
<li *ngFor="let post of posts">{{ post.title }}</li>
</ul>
<div *ngIf="error" class="error-message">
{{ error }}
</div>
`,
styles: [
`
.error-message {
color: red;
margin-top: 10px;
}
`,
],
})
export class ApolloPostListComponent implements OnDestroy {
// ... (component properties and constructor)
fetchPosts() {
this.loading = true
this.error = null
this.posts = []
let query = gql`
query GetPosts($limit: Int) {
posts(limit: $limit) {
id
title
${this.simulateGraphQLError ? "nonExistentField" : ""}
}
}
`
this.apollo
.watchQuery({
query: query,
variables: {
limit: 10,
},
})
.valueChanges.pipe(
takeUntil(this.unsubscribe$),
mergeMap((result) => {
if (this.simulateNetworkError) {
return throwError(
() => new Error("Simulated network error"),
)
}
if (this.simulateUnexpectedError) {
throw new Error("Simulated unexpected error")
}
return of(result)
}),
catchError((error) => {
this.handleError(error)
return of(null)
}),
)
.subscribe({
next: (result: any) => {
if (result) {
this.posts = result.data?.posts || []
}
this.loading = false
this.cdr.detectChanges()
},
error: (error) => this.handleError(error),
complete: () => {
this.loading = false
this.cdr.detectChanges()
},
})
}
// ... (error handling and simulation methods)
}
Wow, would you look at that beauty? 😍 This component is like a finely tuned engine, ready to fetch your posts with the precision of a Swiss watch. Let's break down what's happening here:
- We're using Apollo's watchQuery method to fetch our posts. It's like having a personal assistant who's always on the lookout for the latest data.
- We've got some nifty error simulation methods. It's like having a crash test dummy for your data fetching - you can deliberately cause errors to see how your app handles them. Safety first, right?
- The mergeMap operator is our traffic controller, deciding whether to let the data through or throw an error based on our simulation flags.
- We're using takeUntil with a Subject to ensure we clean up our subscriptions when the component is destroyed. It's like having an eco-friendly car that doesn't leave any pollution (memory leaks) behind!
- The template gives us a simple UI to fetch posts and trigger various error scenarios. It's like having a dashboard with different buttons to test your car's performance.
Error Handling
Speaking of errors, Apollo doesn't just fetch data - it's got your back when things go wrong. Check out this error handling logic:
private handleError(error: any) {
this.loading = false;
if (error.networkError) {
this.error = 'Network error. Please check your internet connection.';
} else if (error.graphQLErrors) {
this.error = `GraphQL error: ${error.graphQLErrors
.map((e: { message: any }) => e.message)
.join(', ')}`;
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
console.error('Error fetching posts', error);
this.cdr.detectChanges();
}
This error handler is like having a built-in mechanic. Whether it's a network issue (like running out of gas) or a GraphQL error (engine trouble), it's got you covered with user-friendly messages.
Wrapping Up Apollo Angular
And there you have it, folks! Apollo Angular - the smooth-riding, feature-packed, error-handling marvel of the GraphQL world. It's like driving a luxury car with a supercomputer onboard.
2. Axios - The Versatile Muscle Car of HTTP Clients
If Apollo Angular is the luxury sports car of GraphQL clients, then Axios is like a classic muscle car - powerful, versatile, and ready to handle anything you throw at it. It might not have all the GraphQL-specific bells and whistles, but boy, can it perform!
1. Installation and Integration Steps
Before we hit the gas, let's get our Axios engine installed and tuned up:
- Installations.
First, rev up your terminal and run:
npm install axios
Unlike Apollo, Axios doesn't need any special configuration in your app.config.ts. It's more of a plug-and-play solution. Just import it where you need it, and you're good to go!
- Code Snippets
Now, below we implement data fetching using axios in src/app/axios-angular/post-list.component.ts
:
import {
Component,
OnInit,
ChangeDetectorRef,
} from "@angular/core"
import {CommonModule} from "@angular/common"
import axios, {AxiosInstance, AxiosError} from "axios"
@Component({
selector: "app-axios-post-list",
standalone: true,
imports: [CommonModule],
template: `
<h2>Posts (Axios Angular)</h2>
<button (click)="fetchPosts()" [disabled]="loading">
{{ loading ? "Loading..." : "Load Posts" }}
</button>
<button (click)="triggerNetworkError()">
Trigger Network Error
</button>
<button (click)="triggerGraphQLError()">
Trigger GraphQL Error
</button>
<button (click)="triggerUnexpectedError()">
Trigger Unexpected Error
</button>
<ul *ngIf="!error">
<li *ngFor="let post of posts">{{ post.title }}</li>
</ul>
<div *ngIf="error" class="error-message">
{{ error }}
</div>
`,
// ... (styles omitted for brevity)
})
export class AxiosPostsListsComponent implements OnInit {
private client: AxiosInstance
posts: any[] = []
loading = false
error: string | null = null
// Error simulation flags
private simulateNetworkError = false
private simulateGraphQLError = false
private simulateUnexpectedError = false
constructor(private cdr: ChangeDetectorRef) {
this.client = axios.create({
baseURL: "/graphql",
headers: {
"Content-Type": "application/json",
},
})
}
ngOnInit() {
// Add a request interceptor
this.client.interceptors.request.use(
(config) => {
if (this.simulateNetworkError) {
return Promise.reject(
new Error("Simulated network error"),
)
}
return config
},
(error) => Promise.reject(error),
)
}
private GET_DATA = `
query GetPosts($limit: Int) {
posts(limit: $limit) {
id
title
${this.simulateGraphQLError ? "nonExistentField" : ""}
}
}
`
async query(queryString: string, variables: any = {}) {
try {
if (this.simulateUnexpectedError) {
throw new Error("Simulated unexpected error")
}
const response = await this.client.post("", {
query: queryString,
variables,
})
return response.data
} catch (error) {
this.handleError(error)
throw error
}
}
async fetchPosts() {
this.loading = true
this.error = null
this.posts = []
this.cdr.detectChanges()
try {
const result = await this.query(this.GET_DATA, {
limit: 10,
})
this.posts = result.data.posts
this.loading = false
this.cdr.detectChanges()
} catch (error) {
// Error is already handled in query method
this.loading = false
this.cdr.detectChanges()
}
}
// ... (error handling and simulation methods omitted for brevity)
}
This Axios-powered component is revving up to fetch those posts faster than you can say "GraphQL"! Let's break down what's happening in this high-octane code:
- We're creating an Axios instance in the constructor. It's like customizing your car with a specific paint job (baseURL) and some cool decals (headers).
- The ngOnInit method adds a request interceptor. Think of it as a nitrous oxide system - it can give your requests an extra boost or, in this case, simulate a network error if you want to test your error handling.
- Our query method is like the engine of this muscle car. It takes a GraphQL query string and variables, then fires off the request. If something goes wrong, it calls our trusty mechanic (the handleError method).
- The fetchPosts method is where the rubber meets the road. It calls our query method with the posts query, then updates our component state with the results.
- We've got our error simulation methods, just like in the Apollo example. It's like having different test tracks for your muscle car - you can simulate various error conditions to make sure your code can handle any bumps in the road.
2. Error Handling
Now, let's talk about handling of errors:
private handleError(error: any) {
if (axios.isAxiosError(error)) {
const axiosError = error as AxiosError;
if (axiosError.response) {
// The request was made and the server responded with a status code
// that falls out of the range of 2xx
this.error = `Server error: ${axiosError.response.status} ${axiosError.response.statusText}`;
} else if (axiosError.request) {
// The request was made but no response was received
this.error = 'Network error. Please check your internet connection.';
} else {
// Something happened in setting up the request that triggered an Error
this.error = 'An unexpected error occurred. Please try again later.';
}
} else if (error.graphQLErrors) {
this.error = `GraphQL error: ${error.graphQLErrors
.map((e: any) => e.message)
.join(', ')}`;
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
console.error('Error fetching posts:', error);
}
This error handler is like the world's best shock absorber system. Whether you hit a pothole (network error), take a wrong turn (server error), or your engine misfires (unexpected error), it's got you covered with user-friendly messages. It even handles those tricky GraphQL-specific errors!
Wrapping Up Axios
And there you have it, Axios - the muscle car of HTTP clients, now tuned up to handle GraphQL queries with style. It might not have all the GraphQL-specific features of Apollo, but it's a powerhouse that can handle just about anything you throw at it. Axios shines when you need a lightweight, versatile solution that can handle both REST and GraphQL APIs. It's like having a car that's equally at home on the racetrack and the city streets. Plus, if you're already familiar with Axios from REST API work, the learning curve here is as smooth as a freshly paved highway.
3. Fetch API - The Lean, Mean, JavaScript Machine
If Apollo was our luxury sports car and Axios our muscle car, then the Fetch API is like a nimble, lightweight motorcycle. It's built right into modern browsers, requires no external libraries, and can zip through traffic with ease. Let's see how this speed demon handles our GraphQL queries!
1. Installation and Integration Steps
Here's the beauty of the Fetch API - there's nothing to install! 🎉 It's like finding out your new apartment comes with a free motorcycle in the garage. Just hop on and ride!
2. Code Snippets
import {Component, ChangeDetectorRef} from "@angular/core"
import {CommonModule} from "@angular/common"
@Component({
selector: "app-fetch-post-list",
standalone: true,
imports: [CommonModule],
template: `
<h2>Posts (Fetch Angular)</h2>
<button (click)="fetchPosts()" [disabled]="loading">
{{ loading ? "Loading..." : "Load Posts" }}
</button>
<button (click)="triggerNetworkError()">
Trigger Network Error
</button>
<button (click)="triggerGraphQLError()">
Trigger GraphQL Error
</button>
<button (click)="triggerUnexpectedError()">
Trigger Unexpected Error
</button>
<ul *ngIf="!error">
<li *ngFor="let post of posts">{{ post.title }}</li>
</ul>
<div *ngIf="error" class="error-message">
{{ error }}
</div>
`,
// ... (styles omitted for brevity)
})
export class FetchPostListComponent {
private endpoint = "/graphql"
posts: any[] = []
loading = false
error: string | null = null
// Error simulation flags
private simulateNetworkError = false
private simulateGraphQLError = false
private simulateUnexpectedError = false
constructor(private cdr: ChangeDetectorRef) {}
private GET_DATA = `
query GetPosts($limit: Int) {
posts(limit: $limit) {
id
title
${this.simulateGraphQLError ? "nonExistentField" : ""}
}
}
`
async query(queryString: string, variables: any = {}) {
if (this.simulateNetworkError) {
throw new Error("Simulated network error")
}
if (this.simulateUnexpectedError) {
throw new Error("Simulated unexpected error")
}
try {
const response = await fetch(this.endpoint, {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
query: queryString,
variables,
}),
})
if (!response.ok) {
throw new Error(
`HTTP error! status: ${response.status}`,
)
}
const result = await response.json()
if (result.errors) {
throw new Error(
result.errors
.map((e: any) => e.message)
.join(", "),
)
}
return result
} catch (error) {
this.handleError(error)
throw error
}
}
async fetchPosts() {
this.loading = true
this.error = null
this.posts = []
this.cdr.detectChanges()
try {
const result = await this.query(this.GET_DATA, {
limit: 10,
})
this.posts = result.data.posts
this.loading = false
this.cdr.detectChanges()
} catch (error) {
// Error is already handled in query method
this.loading = false
this.cdr.detectChanges()
}
}
// ... (error handling and simulation methods omitted for brevity)
}
This Fetch-powered component is leaner than a greyhound and faster than a caffeinated cheetah! Let's break down what's happening in this high-speed code:
- No imports needed for Fetch - it's built right into the browser. It's like having a motorcycle that doesn't need gas!
- Our query method is the engine of this speed machine. It takes a GraphQL query string and variables, then zooms off to fetch the data.
- We're using async/await syntax, which makes our asynchronous code read like a smooth ride down the highway.
- The fetchPosts method is where we kick into high gear. It calls our query method with the posts query, then updates our component state with the results.
- We've still got our error simulation methods. It's like having different obstacle courses for our motorcycle - we can test how it handles in various tricky situations.
Error Handling
Now, let's talk about the suspension system of our Fetch motorcycle - the error handling:
private handleError(error: any) {
if (error instanceof TypeError && error.message === 'Failed to fetch') {
this.error = 'Network error. Please check your internet connection.';
} else if (error instanceof Error) {
if (error.message.includes('GraphQL error')) {
this.error = `GraphQL error: ${error.message}`;
} else if (error.message.startsWith('HTTP error!')) {
this.error = `Server error: ${error.message}`;
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
console.error('Error fetching posts:', error);
}
This error handler efficiently manages various error types. It provides user-friendly messages for network issues, server errors, unexpected problems, and GraphQL-specific errors, ensuring a smooth user experience even when things go wrong.
Wrapping Up Fetch API
And there you have it, The Fetch API - the nimble, lightweight motorcycle of HTTP clients, now revved up to handle GraphQL queries with style. It might not have all the bells and whistles of Apollo or the versatility of Axios, but it's fast, it's built-in, and it gets the job done with minimal fuss.
Fetch shines when you need a lightweight, no-dependency solution that can handle both REST and GraphQL APIs. It's like having a motorcycle that's equally at home zipping through city traffic or cruising on the open highway. Plus, if you're looking to keep your project dependencies to a minimum, Fetch is your go-to ride.
4. GraphQL Request - The Precision-Engineered Sports Car
If Apollo was our luxury sedan, Axios our muscle car, and Fetch our nimble motorcycle, then GraphQL Request is like a finely-tuned sports car. It's designed specifically for GraphQL, offering a perfect balance of simplicity and power. Let's see how this beauty handles our data-fetching curves!
- Installation and Integration Steps Before we hit the track, let's get our GraphQL Request engine installed:
npm install graphql-request graphql
No special configuration needed in your app.config.ts. Just import it in your component, and you're ready to race!
- Code Snippets
Now, let's pop the hood and examine our GraphQL Request-powered component:
import {Component, ChangeDetectorRef} from "@angular/core"
import {CommonModule} from "@angular/common"
import {
GraphQLClient,
gql,
ClientError,
} from "graphql-request"
@Component({
selector: "app-graphql-request-post-list",
standalone: true,
imports: [CommonModule],
template: `
<h2>Posts (Graphql Request Angular)</h2>
<button (click)="fetchPosts()" [disabled]="loading">
{{ loading ? "Loading..." : "Load Posts" }}
</button>
<button (click)="triggerNetworkError()">
Trigger Network Error
</button>
<button (click)="triggerGraphQLError()">
Trigger GraphQL Error
</button>
<button (click)="triggerUnexpectedError()">
Trigger Unexpected Error
</button>
<ul *ngIf="!error">
<li *ngFor="let post of posts">{{ post.title }}</li>
</ul>
<div *ngIf="error" class="error-message">
{{ error }}
</div>
`,
// ... (styles omitted for brevity)
})
export class GraphqlRequestPostListComponent {
private client: GraphQLClient
posts: any[] = []
loading = false
error: string | null = null
// Error simulation flags
private simulateNetworkError = false
private simulateGraphQLError = false
private simulateUnexpectedError = false
constructor(private cdr: ChangeDetectorRef) {
this.client = new GraphQLClient(
"http://localhost:4200/graphql",
)
}
private GET_DATA = gql`
query GetPosts($limit: Int) {
posts(limit: $limit) {
id
title
${this.simulateGraphQLError ? "nonExistentField" : ""}
}
}
`
async fetchPosts() {
this.loading = true
this.error = null
this.posts = []
this.cdr.detectChanges()
try {
if (this.simulateNetworkError) {
throw new Error("Simulated network error")
}
if (this.simulateUnexpectedError) {
throw new Error("Simulated unexpected error")
}
const result: any = await this.client.request(
this.GET_DATA,
{
limit: 10,
},
)
this.posts = result.posts
this.loading = false
this.cdr.detectChanges()
} catch (error) {
this.handleError(error)
this.loading = false
this.cdr.detectChanges()
}
}
// ... (error handling and simulation methods omitted for brevity)
}
Let's break down what's happening in this high-performance code:
- We're importing GraphQLClient and gql from graphql-request. It's like having a custom-built engine and transmission, specifically designed for GraphQL roads.
- In the constructor, we're initializing our GraphQLClient. It's like setting up the onboard computer of our sports car, telling it exactly where to go for our data.
- Our GET_DATA query is defined using the gql tag. It's like programming the GPS with the exact route we want to take.
- The fetchPosts method is where we put the pedal to the metal. We're using the client.request method, which is like engaging the launch control on our sports car - it handles everything for us, from acceleration to gear shifts.
- We've still got our error simulation methods. It's like having different road conditions we can simulate - wet roads, oil slicks, you name it!
Error Handling
Now, let's talk about the advanced traction control system of our GraphQL Request sports car - the error handling:
private handleError(error: any) {
if (error instanceof ClientError) {
if (error.response.errors) {
// GraphQL errors
this.error = `GraphQL error: ${error.response.errors
.map((e) => e.message)
.join(', ')}`;
} else {
// Network errors or other HTTP errors
this.error = `Network error: ${error.response.status} ${error.response['statusText']}`;
}
} else if (error instanceof Error) {
if (error.message === 'Simulated network error') {
this.error = 'Network error. Please check your internet connection.';
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
console.error('Error fetching posts:', error);
}
This error handler is like having the world's best traction control and stability management system. Whether you hit a patch of black ice (network error), take a corner too fast (GraphQL error), or encounter an unexpected obstacle (other errors), it's got you covered with user-friendly messages. It even distinguishes between different types of errors, giving you precise control over how to handle each situation.
Wrapping Up GraphQL Request
And there you have it, folks! GraphQL Request - the precision-engineered sports car of GraphQL clients. It's streamlined, efficient, and designed specifically for the twists and turns of GraphQL queries. GraphQL Request shines when you need a lightweight, GraphQL-specific solution that offers more than Fetch but doesn't require the full ecosystem of Apollo. It's like having a sports car that's perfect for both daily commutes and weekend track days. Plus, its simplicity makes it a joy to work with, especially for smaller to medium-sized projects.
5. Urql in Angular
Installation and Integration Steps
First things first, let's get our hands dirty with some installation magic. To bring Urql into your Angular project, you'll need to wave your command line wand and chant:
npm install @urql/core graphql
We need to set up our Urql client.
Code Snippets and Explanation
Let's break down our UrqlPostListComponent which you'll create following the same format above and solder structure:
import {
createClient,
fetchExchange,
cacheExchange,
Client,
} from "@urql/core"
// ... other imports
export class UrqlPostListComponent {
client: Client
constructor(private cdr: ChangeDetectorRef) {
this.client = createClient({
url: "http://localhost:4200/graphql",
exchanges: [cacheExchange, fetchExchange],
})
}
// ... rest of the component
}
Here, we're setting up our Urql client faster than you can say "GraphQL". We're telling it where to find our GraphQL endpoint and which exchanges to use. Think of exchanges as middleware for your GraphQL requests - they're like bouncers at a club, deciding how to handle incoming and outgoing traffic.
Now, let's look at how we're fetching posts:
getPostsQuery = gql`
query GetPosts($limit: Int) {
posts(limit: $limit) {
id
title
}
}
`;
fetchPosts() {
this.loading = true;
this.error = null;
this.posts = [];
this.cdr.detectChanges();
this.client
.query(this.getPostsQuery, { limit: 10 })
.toPromise()
.then((result) => {
if (result.error) {
this.handleError(result.error);
} else {
this.posts = result.data?.posts || [];
this.loading = false;
this.cdr.detectChanges();
}
})
.catch((error) => this.handleError(error))
.finally(() => {
this.loading = false;
this.cdr.detectChanges();
});
}
This fetchPosts method is where the magic happens. We're using Urql's query method to fetch our posts, handling the result like a pro juggler. If there's an error, we toss it to our error handler. If it's successful, we update our posts faster than you can say "data fetched"!
Error Handling
Now, let's talk about error handling. In the world of APIs, errors are like unexpected plot twists in a movie - they keep things interesting, but you need to know how to handle them:
private handleError(error: any) {
this.loading = false;
if (error instanceof CombinedError) {
if (error.networkError) {
this.error = 'Network error. Please check your internet connection.';
} else if (error.graphQLErrors.length > 0) {
this.error = `GraphQL error: ${error.graphQLErrors
.map((e) => e.message)
.join(', ')}`;
}
} else if (error instanceof Error) {
this.error = `An unexpected error occurred: ${error.message}`;
} else {
this.error = 'An unexpected error occurred. Please try again later.';
}
console.error('Error fetching posts:', error);
this.cdr.detectChanges();
}
This error handler is like a Swiss Army knife for API errors. Network error? We've got you covered. GraphQL error? No problem. Unexpected error that makes you question the nature of reality? We handle that too! Why Choose Urql? You might be wondering, "Why should I choose Urql over other options?" Well, let me tell you, Urql is like that cool, efficient friend who always knows the best way to get things done:
- Lightweight: Urql is as light as a feather, which means your app won't feel like it's carrying extra baggage.
- Flexible: It's adaptable to various use cases, like a chameleon in the coding world.
- Great Developer Experience: With Urql, you'll feel like you're coding with a tailwind, not against a headwind.
Want to see all this in action? Check out our GitHub repo!
We've put together a complete set of working examples for everything we've covered in this article. It's the perfect companion to help you dive deeper into Angular and GraphQL.
Detailed Comparison Table
Method | Bundle Size (minified + gzip)* | Learning Curve | Caching Capabilities | Community Support | Additional Features |
---|---|---|---|---|---|
Apollo Angular¹ | 258 KB | Moderate | Extensive (InMemoryCache, customizable) | High | State management, optimistic UI updates |
Urql² | 17 KB | Low | Moderate (Document and normalized caching) | Moderate | Extensible architecture, lightweight, plugin system |
GraphQL-Request³ | 58.6 KB | Low | None (Minimal client) | Moderate | Simplicity, works in Node and browsers |
Axios⁴ | 24 KB | Low | None (HTTP client only) | High | Familiar HTTP handling, interceptors |
Fetch API | 0 KB (Browser built-in) | Low | None (Native API) | High | No additional dependency, widely supported |
(*) Bundle sizes are based on bundlejs.com calculations using the provided export statements, with minification and gzip compression applied.
Notes:
- Apollo Angular's bundle size (258 KB gzipped) is significantly larger than other options, which may impact initial load times for applications.
- Urql offers a much smaller bundle size (17 KB gzipped) while still providing both document caching and normalized caching through its plugin architecture.
- GraphQL-Request, despite being a minimal client, has a larger bundle size (58.6 KB gzipped) than expected, which might be due to including the full GraphQL parser.
- Axios, a general-purpose HTTP client, has a moderate bundle size (24 KB gzipped) considering its feature set.
- The Fetch API remains the lightest option as it's built into modern browsers, but it lacks some conveniences provided by other libraries.
- Bundle sizes for critical path libraries can significantly impact performance. Consider lazy-loading or code-splitting strategies when using larger libraries like Apollo Angular.
Bundle Size References:
- Apollo Angular: bundlejs.com link
- Urql: bundlejs.com link
- GraphQL-Request: bundlejs.com link
- Axios: bundlejs.com link
Caching Capabilities
-
Apollo Angular
- Extensive caching capabilities through InMemoryCache
- Normalization of data for efficient storage and retrieval
- Customizable cache policies (cache-first, network-only, etc.)
- Automatic cache updates on mutations
- Support for pagination and optimistic UI updates
- Ability to manually update and read from the cache
-
Urql
- Document caching by default
- Customizable caching through exchangeable cache implementations
- Supports normalized caching with additional setup
- Cache invalidation and updates through GraphQL mutations
- Simpler caching model compared to Apollo, focusing on ease of use
-
GraphQL-Request
- No built-in caching mechanism
- Requires manual implementation of caching if needed
- Can be combined with external caching solutions or state management libraries
-
Axios
- No built-in GraphQL-specific caching
- Can implement HTTP-level caching (e.g., using headers)
- Requires manual implementation of application-level caching
- Can be combined with state management libraries for more sophisticated caching
-
Fetch API
- No built-in GraphQL-specific caching
- Supports basic HTTP caching through cache-control headers
- Requires manual implementation of application-level caching
- Can be combined with other libraries or custom solutions for more advanced caching
In summary, Apollo Angular offers the most robust out-of-the-box caching solution, followed by Urql with its flexible caching system. GraphQL-Request, Axios, and Fetch API do not provide GraphQL-specific caching, requiring developers to implement their own caching strategies or integrate with other libraries for advanced caching needs.
When choosing an approach, consider your application's complexity, performance requirements, and willingness to manage caching manually versus leveraging built-in solutions.
Common Issues and Resolutions
-
Apollo Angular
Issue: Cache inconsistencies after mutations Resolution:
- Ensure proper cache updates in mutation's
update
function - Use
refetchQueries
option to refresh related queries - Implement
optimisticResponse
for immediate UI updates
Issue: Over-fetching data Resolution:
- Utilize fragments for reusable field selections
- Implement proper query splitting for components
- Use
@connection
directive for pagination to avoid refetching all data
- Ensure proper cache updates in mutation's
-
Urql
Issue: Stale data after mutations Resolution:
- Use the
cache-and-network
request policy - Implement cache updates in mutation's
updates
option - Utilize the
refocusExchange
for automatic refetching on window focus
Issue: Complex state management Resolution:
- Combine Urql with external state management libraries like NgRx if needed
- Leverage Urql's
useQuery
anduseMutation
hooks for simpler state handling
- Use the
-
GraphQL-Request
Issue: Lack of automatic caching Resolution:
- Implement manual caching using services or state management libraries
- Use HTTP caching headers for basic caching needs
- Consider switching to Apollo or Urql for more complex applications
Issue: Error handling complexities Resolution:
- Implement a centralized error handling service
- Use TypeScript for better type checking and error prevention
- Wrap GraphQL-Request calls in try-catch blocks for granular error handling
-
Axios
Issue: Constructing complex GraphQL queries Resolution:
- Use template literals for dynamic query construction
- Implement a query builder utility for complex queries
- Consider using a GraphQL-specific library for very complex schemas
Issue: Handling GraphQL errors Resolution:
- Check for
errors
array in the response body - Implement custom error classes for different GraphQL error types
- Use interceptors for global error handling
-
Fetch API
Issue: Verbose syntax for GraphQL operations Resolution:
- Create utility functions to abstract common GraphQL operations
- Use TypeScript interfaces for better type safety and autocompletion
- Consider using a lightweight wrapper around Fetch for GraphQL specifics
Issue: Limited built-in features Resolution:
- Implement custom middleware for features like retries and caching
- Use external libraries for advanced features (e.g., Observable support)
- Create a custom Angular service to encapsulate Fetch API logic
General Resolutions:
- Implement proper error boundaries in your Angular components
- Use TypeScript for better type checking and IDE support
- Leverage Angular's HttpInterceptors for global request/response handling
- Implement proper loading states to improve user experience during data fetching
- Use environment variables for GraphQL endpoint configuration
By addressing these common issues, developers can create more robust and efficient GraphQL implementations in their Angular applications, regardless of the chosen approach.
Conclusion
As we've journeyed through the landscape of GraphQL integration in Angular, we've explored five distinct approaches, each with its own strengths and considerations. Let's recap and draw some final insights:
-
Apollo Angular emerges as the powerhouse solution, offering a comprehensive feature set including robust caching, state management, and optimistic UI updates. It's ideal for large-scale applications with complex data requirements, though it comes with a steeper learning curve and larger bundle size.
-
Urql strikes a balance between functionality and simplicity. Its lightweight nature and extensible architecture make it an excellent choice for projects that need flexibility without the full weight of Apollo. It's particularly suitable for medium-sized applications or teams that prefer a more customizable approach.
-
GraphQL-Request shines in its simplicity. For small projects or microservices where basic GraphQL operations are all that's needed, it provides a no-frills solution with minimal overhead. However, it lacks built-in caching and advanced features, which may become limitations as your project grows.
-
Axios, while not GraphQL-specific, leverages its widespread adoption and familiarity among developers. It's a solid choice for teams already using Axios in their stack or for projects that mix RESTful and GraphQL APIs. However, it requires more manual work for GraphQL-specific features.
-
Fetch API represents the most lightweight approach, with zero additional bundle size. It's ideal for projects prioritizing minimal dependencies and maximum browser compatibility. However, it necessitates more boilerplate code and manual implementation of GraphQL-specific features.
The choice between these approaches ultimately depends on your project's specific needs, your team's expertise, and your application's scalability requirements. Here are some final recommendations:
- For large, data-intensive applications with complex requirements, Apollo Angular is likely your best bet.
- If you're looking for a lightweight yet capable solution, Urql offers an excellent middle ground.
- For smaller projects or microservices, GraphQL-Request or Fetch API might be sufficient.
- If your project involves a mix of REST and GraphQL APIs, consider Axios for its versatility.
Remember, there's no one-size-fits-all solution. The best approach is the one that aligns with your project's needs and your team's capabilities. As your application evolves, don't hesitate to reassess and switch approaches if necessary.
Whichever path you choose, GraphQL's power in providing flexible, efficient data fetching can significantly enhance your Angular applications. By understanding these different approaches, you're now equipped to make an informed decision and leverage GraphQL to its full potential in your Angular projects.
Happy coding, and may your GraphQL queries be ever efficient!