GraphQL in Vue: 5 Best Approaches for Data Fetching
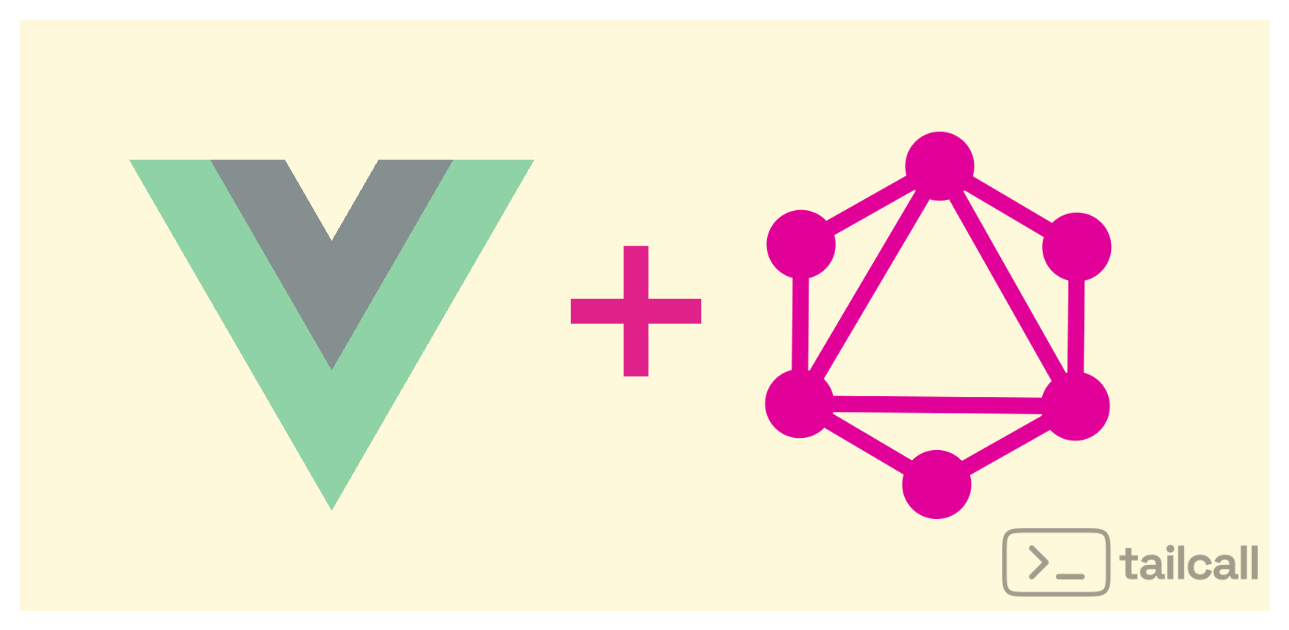
Are you tired of wrestling with complex data fetching logic in your Vue applications? If you've ever felt like you're battling an octopus to retrieve the data you need, then GraphQL is here to be your data fetching hero!
GraphQL empowers you to take control of your data requests in Vue.js, ensuring you receive only the specific data your application requires. This translates to cleaner code, faster performance, and a more delightful developer experience.
In this comprehensive guide, we'll unveil the top 5 approaches to seamlessly integrate GraphQL into your Vue projects. It's like opening a treasure chest overflowing with powerful data fetching techniques!
Whether you're a GraphQL novice or a seasoned pro, this blog post caters to all skill levels. We'll delve into each method, providing in-depth explanations, clear comparisons, and practical error handling strategies. Buckle up and prepare to transform into a data-fetching superhero with the power of GraphQL!
Ready to elevate your Vue development experience? Let's dive in!
๐ ๏ธ Project Setupโ
Let's start by setting up our Vue project with Vite, which provides a faster and leaner development experience:
npm create vite@latest vue-graphql-tailcall-showcase -- --template vue-ts
cd vue-graphql-tailcall-showcase
npm install
This creates a new Vue 3 project with TypeScript support. Now, let's install the necessary dependencies for our GraphQL experiments:
npm install @apollo/client @vue/apollo-composable graphql
npm install @urql/vue
npm install axios
npm install villus
These installations will allow us to explore different GraphQL client options in our Vue application.
๐ง Tailcall Backend Configurationโ
Now, let's set up our Tailcall backend that will wrap the JSONPlaceholder API, providing a GraphQL interface to RESTful data.
First, create a tailcall
directory in the project root:
mkdir tailcall
Then, create a jsonplaceholder.graphql
file in this directory:
# File: tailcall/jsonplaceholder.graphql
schema
@server(port: 8000, hostname: "0.0.0.0")
@upstream(
baseURL: "http://jsonplaceholder.typicode.com"
httpCache: 42
) {
query: Query
}
type Query {
posts: [Post] @http(path: "/posts")
user(id: Int!): User @http(path: "/users/{{.args.id}}")
}
type User {
id: Int!
name: String!
username: String!
email: String!
phone: String
website: String
}
type Post {
id: Int!
userId: Int!
title: String!
body: String!
user: User @http(path: "/users/{{.value.userId}}")
}
This GraphQL schema defines our API structure, mapping RESTful endpoints to GraphQL types and queries.
To start the Tailcall server, you'll need to have Tailcall installed. If you haven't installed it yet, follow the installation instructions from the Tailcall documentation. Once installed, you can start the server with:
tailcall start ./tailcall/jsonplaceholder.graphql
This command starts a GraphQL server on http://localhost:8000
, which will act as a bridge between our Vue application and the JSONPlaceholder API.
With this setup, we're ready to dive into the exciting world of GraphQL in Vue! ๐ Our Tailcall backend provides a perfect playground for exploring different GraphQL client approaches, allowing us to fetch posts and user data with the flexibility and power of GraphQL queries. In the following sections, we'll explore how to leverage this backend with various GraphQL clients in our Vue application. Get ready for some data-fetching magic! โจ
Alright, let's dive into our first approach: Apollo Client! ๐
Apollo Client - The Swiss Army Knife of GraphQLโ
Apollo Client stands out in the GraphQL ecosystem due to its comprehensive feature set, including intelligent caching, real-time updates, and optimistic UI rendering. For Vue developers working on data-intensive applications, Apollo Client provides a sophisticated approach to state management and data fetching.
1. Setting Up Apollo Client in a Vue.js Projectโ
Begin by installing the necessary packages:
npm install @apollo/client @vue/apollo-composable graphql
Configurationโ
Set up Apollo Client in your Vue application:
// src/apollo.ts
import {
ApolloClient,
InMemoryCache,
createHttpLink,
} from "@apollo/client/core"
const httpLink = createHttpLink({
uri: "https://your-graphql-endpoint.com/graphql",
})
export const apolloClient = new ApolloClient({
link: httpLink,
cache: new InMemoryCache(),
defaultOptions: {
watchQuery: {
fetchPolicy: "cache-and-network",
},
},
})
// main.ts
import {createApp, provide, h} from "vue"
import {DefaultApolloClient} from "@vue/apollo-composable"
import App from "./App.vue"
import {apolloClient} from "./apollo"
const app = createApp({
setup() {
provide(DefaultApolloClient, apolloClient)
},
render: () => h(App),
})
app.mount("#app")
This configuration creates an Apollo Client instance with a default in-memory cache and provides it to the entire Vue application.
2. Executing Queries with Apollo Clientโ
Apollo Client provides the useQuery
composable for executing GraphQL queries. Here's an example of fetching a list of posts:
<script setup lang="ts">
import {useQuery} from "@vue/apollo-composable"
import gql from "graphql-tag"
import {computed} from "vue"
interface Post {
id: number
title: string
body: string
user: {
name: string
}
}
const GET_POSTS = gql`
`
const {result, loading, error, refetch} = useQuery<{
posts: Post[]
}>(GET_POSTS, {
limit: 10,
})
const posts = computed(() => result.value?.posts || [])
const fetchPosts = () => {
refetch()
}
</script>
<template>
<div>
<button @click="fetchPosts" :disabled="loading">
Fetch Posts
</button>
<div v-if="loading">Loading...</div>
<ul v-else-if="posts.length">
<li v-for="post in posts" :key="post.id">
{{ post.title }} by {{ post.user.name }}
</li>
</ul>
<div v-else-if="error">Error: {{ error.message }}</div>
</div>
</template>
This example demonstrates:
- Defining a GraphQL query using
gql
tag - Using the
useQuery
composable to manage the query execution - Handling loading, error, and success states
- Implementing a refetch mechanism for manual query execution
3. Mutations and Optimistic Updatesโ
Apollo Client supports GraphQL mutations with optimistic updates for responsive UIs:
<script setup lang="ts">
import {useMutation} from "@vue/apollo-composable"
import gql from "graphql-tag"
import {ref} from "vue"
const CREATE_POST = gql`
`
const {
mutate: createPost,
loading,
error,
} = useMutation(CREATE_POST)
const title = ref("")
const body = ref("")
const submitPost = async () => {
try {
const {data} = await createPost(
{
title: title.value,
body: body.value,
},
{
optimisticResponse: {
createPost: {
__typename: "Post",
id: "temp-id",
title: title.value,
body: body.value,
},
},
update: (cache, {data}) => {
// Update cache logic here
},
},
)
console.log("Post created:", data.createPost)
// Reset form
title.value = ""
body.value = ""
} catch (e) {
console.error("Error creating post:", e)
}
}
</script>
<template>
<form @submit.prevent="submitPost">
<input v-model="title" placeholder="Title" required />
<textarea
v-model="body"
placeholder="Body"
required
></textarea>
<button type="submit" :disabled="loading">
Create Post
</button>
<div v-if="error">Error: {{ error.message }}</div>
</form>
</template>
This example showcases:
- Defining a GraphQL mutation
- Using the
useMutation
composable - Implementing optimistic updates for immediate UI feedback
- Handling form submission and mutation execution
4. Advanced Apollo Client Featuresโ
Caching and Normalizationโ
Apollo Client's normalized cache is a powerful feature for efficient data management:
import {InMemoryCache, makeVar} from "@apollo/client/core"
export const cache = new InMemoryCache({
typePolicies: {
Query: {
fields: {
posts: {
merge(existing, incoming) {
return incoming
},
},
},
},
Post: {
fields: {
isLiked: {
read() {
return likedPostsVar().includes(this.id)
},
},
},
},
},
})
export const likedPostsVar = makeVar<number[]>([])
This setup demonstrates:
- Custom merge functions for query results
- Computed fields based on reactive variables
- Using reactive variables for local state management
Error Handling and Retry Logicโ
Implement robust error handling and retry logic:
import {ApolloLink} from "@apollo/client/core"
import {onError} from "@apollo/client/link/error"
import {RetryLink} from "@apollo/client/link/retry"
const errorLink = onError(
({graphQLErrors, networkError}) => {
if (graphQLErrors)
graphQLErrors.forEach(({message, locations, path}) =>
console.log(
`[GraphQL error]: Message: ${message}, Location: ${locations}, Path: ${path}`,
),
)
if (networkError)
console.log(`[Network error]: ${networkError}`)
},
)
const retryLink = new RetryLink({
delay: {
initial: 300,
max: Infinity,
jitter: true,
},
attempts: {
max: 5,
retryIf: (error, _operation) => !!error,
},
})
const link = ApolloLink.from([
errorLink,
retryLink,
httpLink,
])
This configuration adds comprehensive error logging and automatic retry for failed requests.
5. Performance Optimizationโ
To optimize performance when using Apollo Client with Vue:
-
Implement pagination for large datasets:
<script setup lang="ts">
import {useQuery} from "@vue/apollo-composable"
import gql from "graphql-tag"
import {ref, computed} from "vue"
const GET_POSTS = gql`
`
const limit = ref(10)
const offset = ref(0)
const {result, loading, fetchMore} = useQuery(
GET_POSTS,
() => ({
offset: offset.value,
limit: limit.value,
}),
)
const posts = computed(() => result.value?.posts || [])
const loadMore = () => {
offset.value += limit.value
fetchMore({
variables: {
offset: offset.value,
limit: limit.value,
},
})
}
</script> -
Use fragments for reusable query parts:
import gql from "graphql-tag"
export const POST_FRAGMENT = gql`
fragment PostDetails on Post {
id
title
body
createdAt
}
`
export const GET_POSTS = gql`
${POST_FRAGMENT}
query GetPosts {
posts {
...PostDetails
}
}
` -
Leverage Apollo Client DevTools for performance monitoring and cache inspection.
Conclusionโ
Apollo Client provides a powerful and flexible solution for integrating GraphQL into Vue.js applications. Its advanced features like normalized caching, optimistic updates, and comprehensive error handling make it an excellent choice for complex, data-intensive applications.
Key takeaways:
- Apollo Client offers a robust caching system that optimizes data fetching and management
- The
useQuery
anduseMutation
composables provide a clean API for GraphQL operations - Optimistic updates enable responsive UIs even before server responses
- Advanced features like custom cache policies and reactive variables offer fine-grained control over data management
- Performance optimization techniques such as pagination and fragments are crucial for scalable applications
URQL - A Lightweight GraphQL Client for Modern Web Developmentโ
URQL stands out for its simplicity and modularity, making it an excellent choice for Vue.js projects that require GraphQL integration without the overhead of more complex libraries. Its lightweight nature contributes to faster load times and improved performance, especially in resource-constrained environments.
Key Features of URQL:โ
- Minimal bundle size
- Built-in cache and normalized caching option
- Easy to extend with custom exchanges
- First-class TypeScript support
2. Setting Up URQL in a Vue.js Projectโ
Installationโ
Begin by installing URQL and its Vue integration:
npm install @urql/vue graphql
Configurationโ
Set up the URQL client in your Vue application:
import {createApp} from "vue"
import urql, {createClient} from "@urql/vue"
import App from "./App.vue"
const client = createClient({
url: "https://your-graphql-endpoint.com/graphql",
})
const app = createApp(App)
app.use(urql, client)
app.mount("#app")
This configuration creates an URQL client and integrates it with Vue's plugin system, making it available throughout your application.
3. Executing Queries with URQLโ
URQL provides a useQuery
composable for executing GraphQL queries. Here's an example of fetching a list of posts:
<script setup lang="ts">
import {useQuery} from "@urql/vue"
import {ref, watch} from "vue"
const postsQuery = `
query GetPosts {
posts {
id
title
body
user {
name
}
}
}
`
const {executeQuery, fetching, error, data} = useQuery({
query: postsQuery,
pause: true, // Start paused to allow manual execution
})
const posts = ref([])
const fetchPosts = () => {
executeQuery()
}
watch(data, (newData) => {
if (newData) {
posts.value = newData.posts
}
})
</script>
<template>
<div>
<button @click="fetchPosts" :disabled="fetching">
Fetch Posts
</button>
<div v-if="fetching">Loading...</div>
<ul v-else-if="posts.length">
<li v-for="post in posts" :key="post.id">
{{ post.title }} by {{ post.user.name }}
</li>
</ul>
<div v-else-if="error">Error: {{ error.message }}</div>
</div>
</template>
This example demonstrates how to:
- Define a GraphQL query
- Use the
useQuery
composable to manage the query execution - Handle loading, error, and success states
- Manually trigger the query execution
4. Mutations and State Updatesโ
URQL also supports GraphQL mutations for modifying data. Here's an example of creating a new post:
<script setup lang="ts">
import {useMutation} from "@urql/vue"
import {ref} from "vue"
const createPostMutation = `
mutation CreatePost($title: String!, $body: String!) {
createPost(input: { title: $title, body: $body }) {
id
title
body
}
}
`
const {executeMutation, fetching, error} = useMutation(
createPostMutation,
)
const title = ref("")
const body = ref("")
const createPost = async () => {
const result = await executeMutation({
title: title.value,
body: body.value,
})
if (result.data) {
console.log("Post created:", result.data.createPost)
// Reset form or update local state
title.value = ""
body.value = ""
}
}
</script>
<template>
<form @submit.prevent="createPost">
<input v-model="title" placeholder="Title" required />
<textarea
v-model="body"
placeholder="Body"
required
></textarea>
<button type="submit" :disabled="fetching">
Create Post
</button>
<div v-if="error">Error: {{ error.message }}</div>
</form>
</template>
This example showcases:
- Defining a GraphQL mutation
- Using the
useMutation
composable - Handling form submission and mutation execution
- Managing loading and error states for the mutation
5. Advanced URQL Features and Best Practicesโ
Caching and Normalizationโ
URQL provides a document cache by default, but for more complex applications, you might want to use the normalized cache:
import {
createClient,
dedupExchange,
cacheExchange,
fetchExchange,
} from "@urql/vue"
import {normalizedCache} from "@urql/exchange-graphcache"
const client = createClient({
url: "https://your-graphql-endpoint.com/graphql",
exchanges: [
dedupExchange,
normalizedCache({
keys: {
Post: (data) => data.id,
},
resolvers: {
Query: {
post: (_, args) => ({
__typename: "Post",
id: args.id,
}),
},
},
}),
fetchExchange,
],
})
This setup enables more efficient caching and automatic updates for related queries when mutations occur.
Error Handling and Retry Logicโ
Implement robust error handling and retry logic:
import {retry} from "@urql/exchange-retry"
const client = createClient({
// ... other configuration
exchanges: [
dedupExchange,
cacheExchange,
retry({
retryIf: (error) =>
!!(error.networkError || error.graphQLErrors),
maxNumberAttempts: 3,
}),
fetchExchange,
],
})
This configuration adds automatic retry for network errors or GraphQL errors, improving the resilience of your application.
6. Performance Optimizationโ
To optimize performance when using URQL with Vue:
-
Leverage server-side rendering (SSR) for initial data loading:
import {createClient, ssrExchange} from "@urql/vue"
const ssr = ssrExchange({
isClient: typeof window !== "undefined",
})
const client = createClient({
url: "https://your-graphql-endpoint.com/graphql",
exchanges: [
dedupExchange,
cacheExchange,
ssr,
fetchExchange,
],
}) -
Implement pagination for large datasets:
<script setup lang="ts">
import {useQuery} from "@urql/vue"
import {ref, computed} from "vue"
const postsQuery = `
query GetPosts($limit: Int!, $offset: Int!) {
posts(limit: $limit, offset: $offset) {
id
title
}
}
`
const limit = ref(10)
const offset = ref(0)
const {data, fetching, error} = useQuery({
query: postsQuery,
variables: computed(() => ({
limit: limit.value,
offset: offset.value,
})),
})
const loadMore = () => {
offset.value += limit.value
}
</script> -
Use fragments for reusable query parts:
const PostFragment = `
fragment PostDetails on Post {
id
title
body
createdAt
}
`
const postsQuery = `
${PostFragment}
query GetPosts {
posts {
...PostDetails
}
}
`
Conclusionโ
URQL provides a lightweight yet powerful solution for integrating GraphQL into Vue.js applications. Its simplicity, coupled with advanced features like normalized caching and SSR support, makes it an excellent choice for developers seeking efficiency and flexibility. By following the best practices and optimization techniques outlined in this tutorial, you can build performant, scalable Vue applications with GraphQL.
Key takeaways:
- URQL offers a minimal bundle size and easy integration with Vue.js
- The
useQuery
anduseMutation
composables provide a clean API for GraphQL operations - Advanced features like normalized caching and SSR support enhance application performance
- Proper error handling and retry logic improve application resilience
- Performance optimization techniques such as pagination and fragments are crucial for scalable applications
As you implement URQL in your Vue projects, remember to stay updated with the latest developments in both URQL and the GraphQL ecosystem to leverage new features and best practices as they emerge.
Fetch API - The DIY Dynamoโ
In modern web development, effective data fetching is a cornerstone for building dynamic and responsive applications. While powerful libraries like Apollo and URQL offer extensive features for GraphQL integration, there are situations where a more hands-on approach is desirable. Enter the Fetch API: a versatile, built-in tool for making network requests, allowing developers to craft their GraphQL interactions from the ground up. This tutorial will guide you through using the Fetch API for GraphQL data fetching in Vue.js, providing a deep understanding of the process and its practical applications.
Step-by-Step Instructionsโ
Installation and Integration Stepsโ
One of the key advantages of the Fetch API is its built-in availability in modern browsers. This means no additional package installations are required, simplifying the setup process.
Code Snippetsโ
Let's start by setting up a basic Vue component that uses the Fetch API to query a GraphQL endpoint.
<template>
<div class="fetch-example">
<h2>Fetch API Example</h2>
<button @click="fetchPosts" :disabled="loading">
Fetch Posts
</button>
<div v-if="networkError" class="error">
Network Error: {{ networkError }}
</div>
<div v-if="graphqlError" class="error">
GraphQL Error: {{ graphqlError }}
</div>
<div v-if="unexpectedError" class="error">
Unexpected Error: {{ unexpectedError }}
</div>
<ul>
<li v-for="post in posts" :key="post.id">
{{ post.title }} by {{ post.user.name }}
</li>
</ul>
</div>
</template>
<script setup lang="ts">
import {ref} from "vue"
interface Post {
id: number
title: string
body: string
user: {
name: string
}
}
const posts = ref<Post[]>([])
const loading = ref(false)
const networkError = ref<string | null>(null)
const graphqlError = ref<string | null>(null)
const unexpectedError = ref<string | null>(null)
const fetchPosts = async () => {
loading.value = true
networkError.value = null
graphqlError.value = null
unexpectedError.value = null
try {
const response = await fetch("/graphql", {
method: "POST",
headers: {"Content-Type": "application/json"},
body: JSON.stringify({
query: `
query GetPosts {
posts {
id
title
body
user {
name
}
}
}
`,
}),
})
if (!response.ok) {
throw new Error(
`HTTP error! status: ${response.status}`,
)
}
const result = await response.json()
if (result.errors && result.errors.length > 0) {
graphqlError.value = result.errors
.map((e: any) => e.message)
.join(", ")
} else {
posts.value = result.data.posts.slice(0, 4)
}
} catch (err: any) {
if (err.message.startsWith("HTTP error!")) {
networkError.value = err.message
} else {
unexpectedError.value = err.message
}
} finally {
loading.value = false
}
}
</script>
Error Handlingโ
Handling errors effectively is crucial in any data-fetching scenario. The above code includes a robust error-handling system that categorizes errors into network errors, GraphQL errors, and unexpected errors.
try {
// ... fetch logic ...
} catch (err: any) {
if (err.message.startsWith("HTTP error!")) {
networkError.value = err.message
} else {
unexpectedError.value = err.message
}
} finally {
loading.value = false
}
This approach ensures that your application can gracefully handle and display errors, enhancing user experience.
Industry Best Practices and Potential Technical Challengesโ
Best Practicesโ
- Modular Code: Break down your Fetch API logic into reusable functions or composables to promote code reusability and maintainability.
- Error Handling: Implement comprehensive error handling to manage different types of errors (network, GraphQL, unexpected) effectively.
- Security: Always validate and sanitize input data, especially when constructing GraphQL queries dynamically.
Technical Challengesโ
- Verbose Error Handling: Handling errors manually can be verbose and requires meticulous coding to cover all edge cases.
- Scalability: For larger projects, the Fetch API might lack the convenience features offered by dedicated GraphQL clients, such as caching and automatic batching.
- State Management: Managing loading states and errors can become complex, especially as the application grows.
Real-World Applicationsโ
Professional Development Scenariosโ
- Custom Solutions: In scenarios where fine-grained control over GraphQL requests is needed, such as specific headers or request configurations, the Fetch API shines.
- Learning and Prototyping: Using the Fetch API is a great way to learn the basics of GraphQL and network requests, making it suitable for educational purposes and prototyping.
- Lightweight Applications: For smaller applications or micro-frontends where adding a full-fledged GraphQL client is overkill, the Fetch API provides a lightweight alternative.
Comparison to Alternative Technologiesโ
Apollo Clientโ
- Pros: Robust, feature-rich, automatic caching, extensive community support.
- Cons: Heavier bundle size, additional dependencies, potentially overkill for simple use cases.
URQLโ
- Pros: Lightweight, flexible, built-in support for common GraphQL patterns.
- Cons: Less mature than Apollo, fewer built-in features.
Axiosโ
- Pros: Versatile HTTP client, can be used with GraphQL, additional features like request cancellation.
- Cons: Requires additional configuration for GraphQL, less intuitive compared to dedicated GraphQL clients.
Summary of Key Technical Insightsโ
- Control and Flexibility: The Fetch API offers unmatched control over GraphQL requests, making it ideal for custom solutions.
- Built-In Availability: No need for additional dependencies, simplifying the development and deployment process.
- Error Handling: Robust error handling is crucial to manage different types of errors effectively.
- Learning Opportunity: Using the Fetch API provides a deeper understanding of GraphQL and network requests, beneficial for both entry-level and intermediate developers.
Conclusionโ
The Fetch API serves as a powerful tool for developers looking to craft their GraphQL solutions with precision and control. While it may not offer the convenience and features of dedicated GraphQL clients, it provides a lightweight and versatile alternative. By following best practices and understanding potential challenges, developers can effectively leverage the Fetch API for various professional development scenarios, gaining valuable insights and experience in the process.
Axios - The Smooth Operatorโ
In the world of HTTP clients, Axios is like a sous chef in your kitchen, handling the tedious tasks and ensuring your GraphQL requests are prepared with finesse. Unlike the Fetch API, Axios simplifies and streamlines data fetching, making it an attractive option for developers seeking efficiency without sacrificing control. Let's dive into how Axios can enhance your Vue.js application with smooth and efficient GraphQL data fetching.
1. Installation and Integration Stepsโ
First, we need to install Axios in our Vue project. This can be done quickly via npm:
npm install axios
That's it! Axios is now ready to use, providing a smooth and easy setup process.
2. Code Snippetsโ
Here's how you can use Axios to fetch data from a GraphQL endpoint in a Vue component:
<script setup lang="ts">
import {ref} from "vue"
import axios from "axios"
interface Post {
id: number
title: string
body: string
user: {
name: string
}
}
const posts = ref<Post[]>([])
const loading = ref(false)
const networkError = ref<string | null>(null)
const graphqlError = ref<string | null>(null)
const unexpectedError = ref<string | null>(null)
const fetchPosts = async () => {
loading.value = true
networkError.value = null
graphqlError.value = null
unexpectedError.value = null
try {
const response = await axios.post("/graphql", {
query: `
query GetPosts {
posts {
id
title
body
user {
name
}
}
}
`,
})
if (response.status !== 200) {
throw new Error(
`HTTP error! status: ${response.status}`,
)
}
const result = response.data
if (result.errors && result.errors.length > 0) {
graphqlError.value = result.errors
.map((e: any) => e.message)
.join(", ")
} else {
posts.value = result.data.posts.slice(0, 4)
}
} catch (err: any) {
if (err.response) {
networkError.value = `HTTP error! status: ${err.response.status}`
} else {
unexpectedError.value = err.message
}
} finally {
loading.value = false
}
}
</script>
<template>
<div class="axios-example">
<h2>Axios API Example</h2>
<button @click="fetchPosts" :disabled="loading">
Fetch Posts
</button>
<div v-if="networkError" class="error">
Network Error: {{ networkError }}
</div>
<div v-if="graphqlError" class="error">
GraphQL Error: {{ graphqlError }}
</div>
<div v-if="unexpectedError" class="error">
Unexpected Error: {{ unexpectedError }}
</div>
<ul>
<li v-for="post in posts" :key="post.id">
{{ post.title }} by {{ post.user.name }}
</li>
</ul>
</div>
</template>
Axios simplifies the process of making GraphQL requests, handling much of the boilerplate code associated with Fetch API. This makes your code cleaner and easier to maintain.
3. Error Handlingโ
Axios provides robust error handling capabilities, making it easier to manage different types of errors:
try {
// ... axios request ...
} catch (err: any) {
if (err.response) {
networkError.value = `HTTP error! status: ${err.response.status}`
} else {
unexpectedError.value = err.message
}
} finally {
loading.value = false
}
This error-handling structure allows you to neatly categorize and handle various error scenarios, ensuring your application remains robust and user-friendly.
4. Why Axios Rocksโ
-
Automatic Transforms: Axios automatically transforms your response data, allowing for smoother data handling. It's like having a translator who speaks both JSON and JavaScript fluently.
-
Request and Response Interceptors: Need to add an auth token to every request or log all responses? Axios interceptors handle these tasks effortlessly, acting like a gatekeeper for your requests.
-
Browser and Node.js Support: Axios works seamlessly in both browser and Node.js environments, providing flexibility for different project requirements.
-
Cancellation Support: Axios allows you to cancel requests, providing an "undo" button for your API calls. This is particularly useful for handling stale or unnecessary requests.
Industry Best Practices and Potential Technical Challengesโ
Best Practicesโ
- Use Interceptors: Leverage Axios interceptors to manage repetitive tasks like setting headers or logging requests and responses.
- Error Handling: Implement comprehensive error handling to ensure your application can gracefully recover from various error conditions.
- Modular Code: Organize your Axios requests into reusable functions or composables to keep your codebase clean and maintainable.
Technical Challengesโ
- Bundle Size: Although Axios is relatively lightweight, it's still an additional dependency that can increase your project's bundle size.
- Customization: While Axios is highly customizable, it may require additional setup compared to more specialized GraphQL clients.
Real-World Applicationsโ
Professional Development Scenariosโ
- API Integration: Axios is ideal for integrating with RESTful and GraphQL APIs, providing a consistent and efficient approach for data fetching.
- Middleware Implementation: Use Axios interceptors to implement middleware for logging, authentication, or other cross-cutting concerns.
- Server-Side Rendering (SSR): With its support for both browser and Node.js environments, Axios is well-suited for SSR applications, ensuring consistent data fetching across platforms.
Summary of Key Technical Insightsโ
- Efficiency and Convenience: Axios offers a balance of control and convenience, making it easier to manage GraphQL requests with minimal boilerplate.
- Robust Error Handling: Built-in error handling features help manage network and unexpected errors effectively.
- Flexibility: With support for both browser and Node.js environments, Axios is versatile and adaptable to various project needs.
- Interceptors and Cancellation: Advanced features like request/response interceptors and request cancellation provide additional control and flexibility.
Conclusionโ
Axios stands out as a versatile and efficient tool for handling GraphQL requests in Vue.js applications. Its combination of automatic data transformation, robust error handling, and advanced features like interceptors and cancellation support make it a powerful choice for developers seeking a smooth and streamlined data-fetching experience. By incorporating best practices and understanding potential challenges, developers can leverage Axios to build robust and responsive applications, gaining valuable insights and experience in the process.
Stay tuned as we continue our journey through the world of GraphQL data fetching in Vue.js. Our final approach will bring everything together, providing the ultimate guide to mastering GraphQL in your Vue applications.
Villus - The Vue-Native Virtuosoโ
For the grand finale of our GraphQL journey, let's introduce Villus! ๐ญ If our previous approaches were a warm-up, Villus is the show-stopping final act. It's a Vue-native GraphQL client that makes your data fetching seamless and elegant, tailored perfectly for Vue.js applications.
1. Installation and Integration Stepsโ
First, let's set up Villus in your Vue project:
npm install villus graphql
Next, configure Villus in your main.js
file:
import {createApp} from "vue"
import {createClient as createVillusClient} from "villus"
import App from "./App.vue"
const villusClient = createVillusClient({
url: "/graphql",
})
const app = createApp(App)
app.use(villusClient)
app.mount("#app")
2. Code Snippetsโ
Here's how to use Villus to fetch data from a GraphQL endpoint in a Vue component:
<script setup lang="ts">
import {useQuery} from "villus"
import {ref} from "vue"
interface Post {
id: number
title: string
body: string
user: {
name: string
}
}
interface QueryResult {
posts: Post[]
}
const posts = ref<Post[]>([])
const loading = ref(false)
const networkError = ref<string | null>(null)
const graphqlError = ref<string | null>(null)
const unexpectedError = ref<string | null>(null)
const query = useQuery<QueryResult>({
query: `
query GetPosts {
posts {
id
title
body
user {
name
}
}
}
`,
paused: true,
})
const fetchPosts = async () => {
loading.value = true
networkError.value = null
graphqlError.value = null
unexpectedError.value = null
try {
const result = await query.execute()
if (result.error) {
throw result.error
}
if (result.data && result.data.posts) {
posts.value = result.data.posts.slice(0, 4)
} else {
throw new Error("Posts not found in query result")
}
} catch (e: any) {
if (e.message.startsWith("Network Error")) {
networkError.value = e.message
} else if (e.graphQLErrors) {
graphqlError.value = e.graphQLErrors
.map((err: any) => err.message)
.join(", ")
} else {
unexpectedError.value = e.message
}
} finally {
loading.value = false
}
}
</script>
<template>
<div class="container">
<h2>Villus Example</h2>
<button @click="fetchPosts" :disabled="loading">
Fetch Posts
</button>
<div v-if="networkError" class="error">
Network Error: {{ networkError }}
</div>
<div v-if="graphqlError" class="error">
GraphQL Error: {{ graphqlError }}
</div>
<div v-if="unexpectedError" class="error">
Unexpected Error: {{ unexpectedError }}
</div>
<ul>
<li v-for="post in posts" :key="post.id">
{{ post.title }} by {{ post.user.name }}
</li>
</ul>
</div>
</template>
Villus integrates seamlessly with Vue, providing a smooth and elegant way to handle GraphQL queries.
3. Error Handlingโ
Villus comes with robust error handling, making it easy to manage various error scenarios:
try {
const result = await query.execute()
if (result.error) {
throw result.error
}
// ... handle successful result ...
} catch (e: any) {
if (e.message.startsWith("Network Error")) {
networkError.value = e.message
} else if (e.graphQLErrors) {
graphqlError.value = e.graphQLErrors
.map((err: any) => err.message)
.join(", ")
} else {
unexpectedError.value = e.message
}
}
This error-handling structure ensures that your application can gracefully recover from various issues, providing a robust user experience.
4. Why Villus Rocksโ
- Vue-Native: Villus is built specifically for Vue, making it a perfect fit for Vue applications. It's like having a custom-tailored suit for your Vue app.
- Lightweight: Villus is lightweight and efficient, ensuring that your application remains fast and responsive.
- Composable: With its composition API support, Villus integrates seamlessly with Vue 3, enhancing the development experience.
- Smart Defaults: Villus comes with smart defaults that work out of the box, but it is also highly customizable to fit your specific needs.
Industry Best Practices and Potential Technical Challengesโ
Best Practicesโ
- Use the Composition API: Leveraging Vue's composition API with Villus makes your code more modular and maintainable.
- Error Handling: Implement comprehensive error handling to ensure a robust application.
- Modular Code: Organize your Villus queries into reusable functions or composables to keep your codebase clean.
Technical Challengesโ
- Learning Curve: For developers new to GraphQL or the composition API, there might be a learning curve.
- Customization: While Villus is highly customizable, it may require additional setup for more complex use cases.
Real-World Applicationsโ
Professional Development Scenariosโ
- API Integration: Villus is perfect for integrating with GraphQL APIs in Vue applications, providing a seamless data fetching experience.
- State Management: Villus can be used alongside Vue's reactive state management, providing a powerful combination for managing application state.
- Server-Side Rendering (SSR): With its support for both client-side and server-side rendering, Villus ensures consistent data fetching across platforms.
Summary of Key Technical Insightsโ
- Efficiency and Convenience: Villus offers a balance of efficiency and convenience, making it easy to manage GraphQL requests in Vue applications.
- Robust Error Handling: Built-in error handling features help manage network and unexpected errors effectively.
- Vue-Native Integration: Villus is built specifically for Vue, providing seamless integration and enhancing the development experience.
- Lightweight and Composable: Villus is lightweight and leverages the composition API, making it a powerful tool for modern Vue development.
Conclusionโ
Villus stands out as a versatile and efficient tool for handling GraphQL requests in Vue.js applications. Its Vue-native design, robust error handling, and lightweight nature make it an excellent choice for developers seeking a seamless data-fetching experience. By incorporating best practices and understanding potential challenges, developers can leverage Villus to build robust and responsive applications, gaining valuable insights and experience in the process.
With this, we've completed our exploration of various data-fetching approaches in Vue. Each approach offers unique strengths and is suited for different scenarios, providing you with the tools and knowledge to choose the best solution for your projects. Happy coding! ๐
Comparison Tableโ
Feature | Apollo Client | URQL | Fetch API | Axios | Villus |
---|---|---|---|---|---|
Bundle Size (Minified + Gzipped)* | 47.8 kB | 10.2 kB | 2.8kB | 13.2kB | 4.6kB |
Learning Curve | Steep | Moderate | Low | Low | Moderate |
Caching Capabilities | Advanced | Good | Manual | Manual | Good |
Community Support | Extensive | Growing | Widespread | Extensive | Limited |
Additional Features | Rich ecosystem, dev tools, local state management | Lightweight, customizable | Native browser API | Request/response interceptors, automatic transforms | Vue-specific, lightweight |
*Bundle sizes culled from bundlephobia.com
Here's a brief explanation of the ratings:
-
Learning Curve:
- Apollo Client: Steep due to its extensive features and concepts.
- URQL: Moderate as it's simpler than Apollo but still has GraphQL-specific concepts.
- Fetch API: Low as it's a basic browser API.
- Axios: Low as it's straightforward to use for HTTP requests.
- Villus: Moderate as it's Vue-specific but simpler than Apollo.
-
Caching Capabilities:
- Apollo Client: Advanced with sophisticated normalization and cache policies.
- URQL: Good built-in caching with customizable options.
- Fetch API: Manual caching required.
- Axios: Manual caching required.
- Villus: Good basic caching capabilities.
-
Community Support:
- Apollo Client: Extensive due to its popularity in the GraphQL ecosystem.
- URQL: Growing community, but not as large as Apollo's.
- Fetch API: Widespread as it's a web standard.
- Axios: Extensive due to its popularity for HTTP requests.
- Villus: Limited as it's a newer and more niche library.
-
Additional Features:
- Apollo Client: Rich ecosystem with developer tools and local state management.
- URQL: Lightweight and highly customizable.
- Fetch API: Native browser API, no extra features.
- Axios: Request/response interceptors, automatic transforms for data.
- Villus: Vue-specific integration, lightweight alternative to Apollo.
This table provides a high-level comparison of the different approaches for GraphQL data fetching in Vue. Each approach has its strengths and may be more suitable depending on the specific requirements of a project.
Caching Capabilitiesโ
-
Apollo Client:
- Offers a sophisticated normalized cache
- Supports various cache policies (cache-first, network-only, etc.)
- Allows for fine-grained cache updates and invalidation
- Provides optimistic UI updates
-
URQL:
- Implements a document cache by default
- Supports customizable caching strategies
- Offers a normalized cache through the Normalized Cache exchange
- Provides easy cache updates and invalidation
-
Fetch API:
- No built-in caching mechanism
- Requires manual implementation of caching logic
- Can leverage browser's HTTP cache or custom in-memory/storage solutions
-
Axios:
- No built-in caching mechanism for GraphQL
- Requires manual implementation of caching logic
- Can be combined with external caching libraries
-
Villus:
- Provides a simple document cache
- Supports cache policies similar to Apollo (cache-first, network-only)
- Offers manual cache manipulation methods
- Lighter weight caching compared to Apollo
Common Issues and Resolutionsโ
-
Apollo Client:
- Issue: Over-fetching data Resolution: Use fragments and optimized queries
- Issue: Cache inconsistencies Resolution: Manually update cache or use refetchQueries
- Issue: Performance with large datasets Resolution: Implement pagination or infinite scrolling
-
URQL:
- Issue: Lack of advanced caching features out-of-the-box Resolution: Use additional exchanges like the Normalized Cache exchange
- Issue: Limited dev tools compared to Apollo Resolution: Rely on browser network tab or implement custom logging
- Issue: Learning curve for exchanges concept Resolution: Start with basic setup and gradually add exchanges as needed
-
Fetch API:
- Issue: Verbose syntax for GraphQL requests Resolution: Create utility functions to simplify request creation
- Issue: No built-in error handling for GraphQL errors Resolution: Implement custom error checking and handling logic
- Issue: Manual caching and state management Resolution: Use state management libraries like Vuex or Pinia alongside Fetch
-
Axios:
- Issue: Not GraphQL-specific, requiring more boilerplate Resolution: Create custom wrapper functions for GraphQL operations
- Issue: Handling GraphQL errors separately from HTTP errors Resolution: Implement middleware to check for and handle GraphQL-specific errors
- Issue: No built-in caching for GraphQL queries Resolution: Implement custom caching layer or use with a state management solution
-
Villus:
- Issue: Limited ecosystem compared to Apollo Resolution: Combine with other Vue libraries for missing features
- Issue: Less community resources and examples Resolution: Refer to official documentation and reach out to maintainers for support
- Issue: Potential performance issues with larger applications Resolution: Consider using more robust solutions like Apollo for very complex apps
Each approach has its own set of challenges, but also offers unique advantages. The choice between them often depends on the specific requirements of your project, the complexity of your GraphQL operations, and your team's familiarity with the tools.
The Grand Finaleโ
We've journeyed through the land of GraphQL in Vue, exploring five fantastic approaches:
- Apollo Client: The Swiss Army knife
- URQL: The lightweight contender
- Fetch API: The DIY dynamo
- Axios: The smooth operator
- Villus: The Vue-native virtuoso
Code snippets used in this article can be found in the GitHub repository. Feel free to explore, experiment, and adapt them to your projects!
Each approach has its own strengths, like instruments in an orchestra. The choice depends on your project's needs, your team's expertise, and the symphony you want to create.
Remember, in the world of web development, there's no one-size-fits-all solution. It's about finding the right tool for your unique masterpiece. So go forth, experiment, and may your Vue apps be forever data-rich and performant!
And with that, we bring down the curtain on our GraphQL in Vue extravaganza. But don't worry, the show never really ends in the ever-evolving world of web development. Keep learning, keep coding, and most importantly, keep having fun! ๐ญ๐