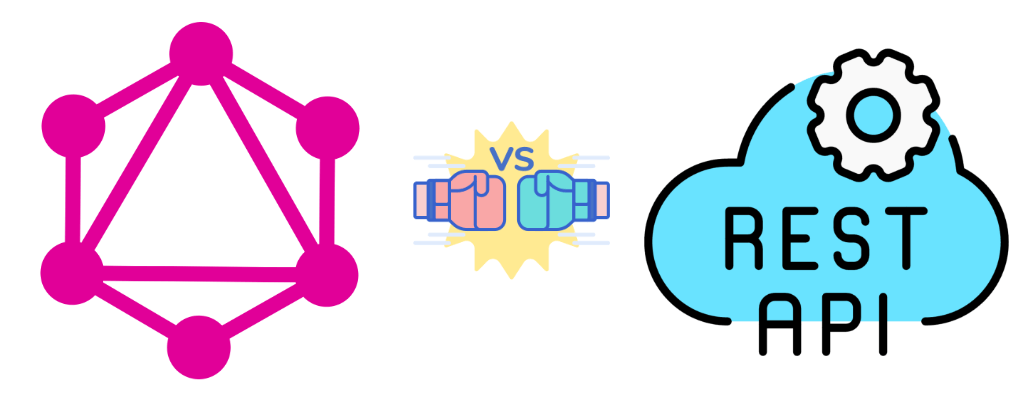
GraphQL vs REST
Is GraphQL Better Than REST? GraphQL vs REST Comparison
In today’s fast-paced digital world, the right API architecture is crucial for the success of web and mobile applications. APIs, or Application Programming Interfaces, are the backbone of data exchange on the internet. They enable different systems to communicate and share data seamlessly.
Among the various API design paradigms, GraphQL and REST stand out as two predominant technologies. This comprehensive guide explores these technologies, highlighting their advantages, disadvantages, and ideal use cases to determine which is better suited for specific project needs.
API design choices have significant implications on the performance, scalability, and maintainability of your applications. Understanding the fundamental differences between GraphQL and REST can help you make informed decisions that align with your project requirements and long-term goals.
What is REST?
REST (Representational State Transfer) is an architectural style for designing networked applications. It uses HTTP requests to perform CRUD (Create, Read, Update, Delete) operations. Each resource in a RESTful system is identified by a unique URI and can be manipulated using standard HTTP methods: GET, POST, PUT, DELETE. It is designed to utilize the stateless operations of the HTTP protocol for web communications. REST has been widely adopted due to its simplicity and effectiveness in designing scalable web services. Detailed Example: Consider a typical social media platform:
GET /users // Retrieves a list of users
POST /users // Creates a new user
GET /users/123 // Retrieves details about user 123
GET /users/123/posts // Retrieves posts by user 123
GET /users/123/followers // Retrieves followers of user 123
GET /posts // Retrieves posts
POST /posts // Creates a post
GET /posts/123/comments // Retrieves comments for post 123
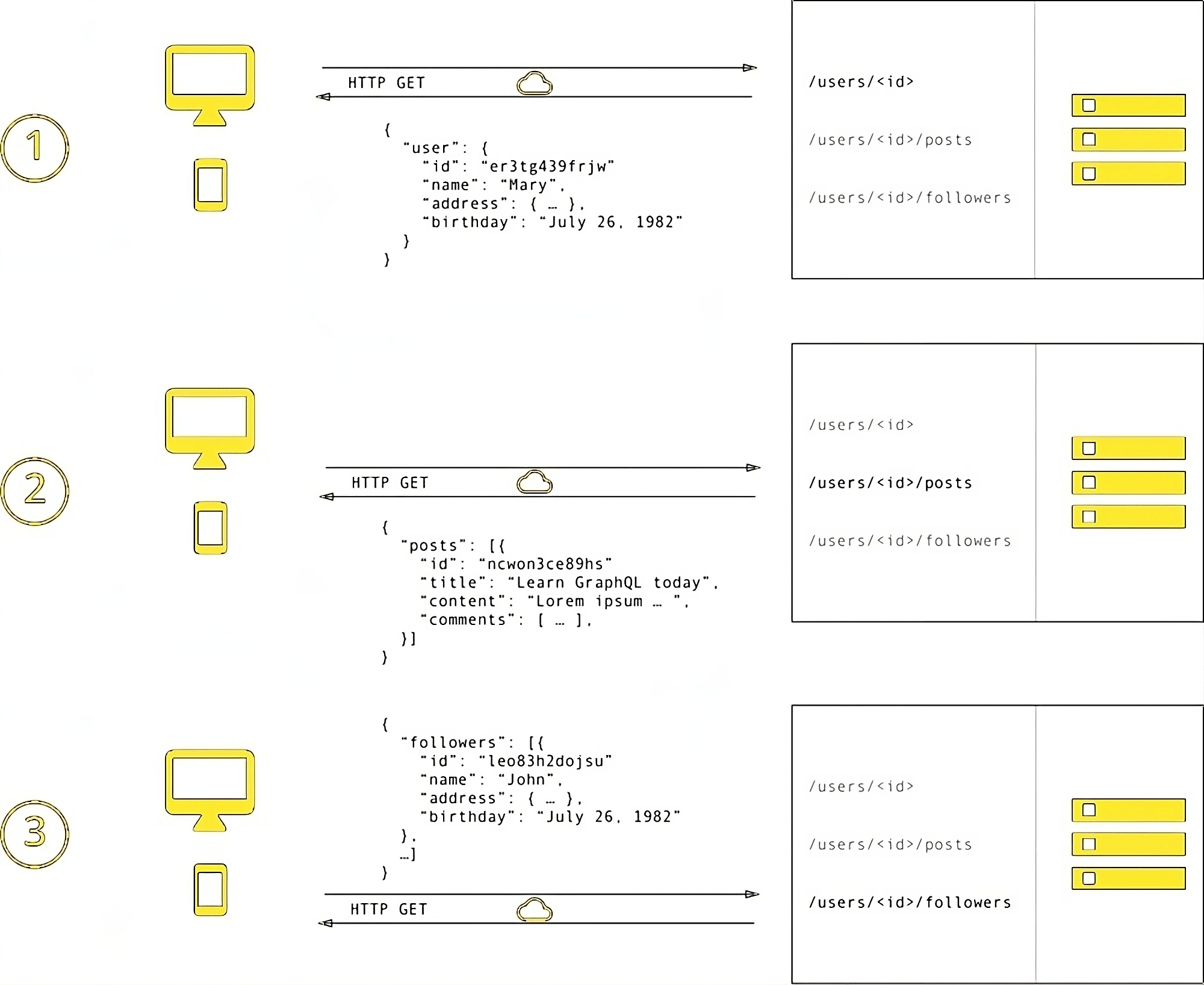
REST API Design
Each endpoint is designed to handle a specific type of resource and HTTP method (GET, POST, PUT, DELETE). This design allows clients to interact with the server in a predictable manner.
Key Characteristics of REST:
- Stateless Operations: Each API request from a client to server must contain all the information needed to understand and process the request. The server side should not store any client context between requests, which is beneficial for scalability and reliability.
- Cacheable: Responses must explicitly define themselves as cacheable or non cacheable. If a response is defined as cacheable, the client can reuse the response for similar requests in future. Server also defines when to invalidate the cached response for a particular request to prevent clients from reusing stale or inappropriate data. A good caching practice can significantly reduce the number of requests to the server.
- Layered System: A client cannot ordinarily tell whether it is connected directly to the end server or an intermediary along the way. A REST API can consist of multiple layers of servers, each with its own specific functionality. For example, an E-Commerce application might have a server for authentication, another for product information, and a third for payment processing. A client can interact with a layer without knowing the details of other layers.
Advantages of REST
- Simplicity and Maturity: REST has been widely used and is supported by most platforms and programming languages (Frontend and Backend), making it a mature choice for building APIs. Its principles are well-understood, and there is a wealth of documentation and tooling available.
- Scalability: Due to its stateless nature and ability to handle requests independently, REST can scale effectively. Each request is treated as an independent transaction, allowing for easy distribution across multiple servers.
- Caching: REST can efficiently leverage web infrastructure for caching requests, reducing the load on the backend and improving performance. HTTP caching mechanisms, such as ETags and cache-control headers, can be used to cache responses and minimize redundant data transfers.
What is GraphQL?
GraphQL is a query language for APIs and a runtime for executing those queries. It allows clients to request exactly the data they need, avoiding over-fetching and under-fetching issues common with REST. Developed by Facebook in 2012 and open-sourced in 2015, GraphQL provides a more flexible and efficient approach to API design.
Key Characteristics of GraphQL:
- Strongly Typed: GraphQL APIs are defined by a schema using the GraphQL Schema Definition Language (SDL).
- Single Endpoint: GraphQL APIs have a single endpoint, making it simpler to manage than multiple REST endpoints. This single endpoint can handle a wide variety of queries and mutations, streamlining the client-server interaction.
- Declarative Data Fetching: Clients can specify exactly what data they need, even to the level of specifying individual fields in a single query. This allows for highly efficient data fetching and reduces the likelihood of over-fetching or under-fetching. Example Query:
{
user(id: "1") {
name
email
posts {
title
comments {
content
author {
name
}
}
}
}
}
In this example, the client requests specific fields (name, email, posts, comments) in a single query, avoiding unnecessary data transfers.
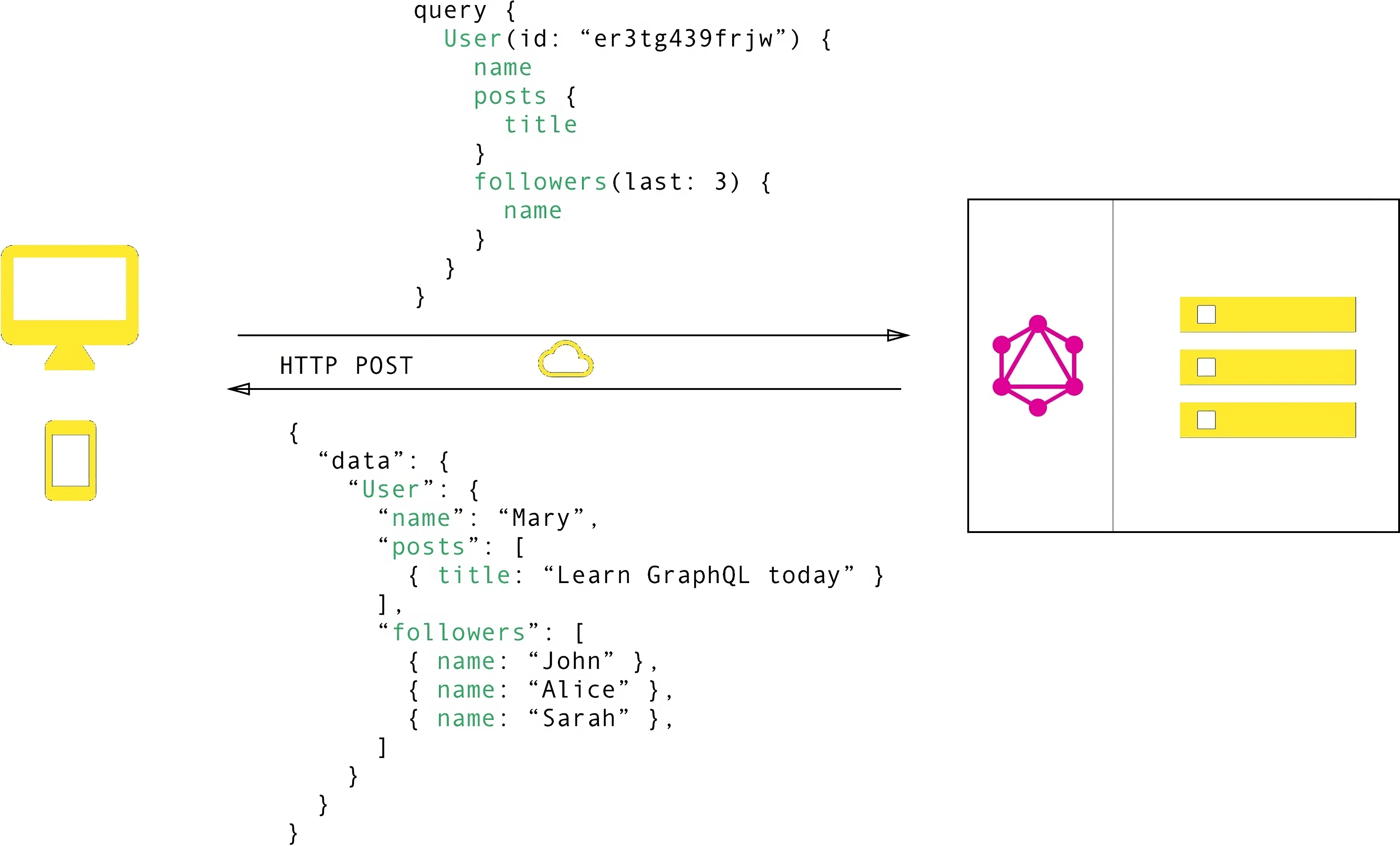
GraphQL Efficiency: This image shows how clients specify data needs, ensuring the server response matches the query structure precisely.
Advantages of GraphQL
- Efficient Data Loading: Reduces over-fetching and under-fetching, leading to more efficient data transfers. Clients can request exactly the data they need, minimizing network traffic and improving performance.
- Strongly Typed: Every GraphQL service defines a set of types which completely describe the set of possible data you can query on that service. This strong typing helps prevent errors and improves the development experience.
- Rapid Development: GraphQL's declarative nature and strong type system make it easier to develop and maintain APIs. The self-documenting nature of GraphQL schemas also aids in understanding the API structure.
Limitations of GraphQL
- Complexity in Client: The flexibility of GraphQL requires clients to handle more complexity in data handling and state management. Clients must be capable of constructing complex queries and managing the resulting data structures.
- Caching: Unlike REST, which can leverage HTTP caching mechanisms, caching a GraphQL API can be more involved because each query can be unique. This requires more sophisticated caching strategies, such as field-level caching or custom cache management solutions.
Similarities Between GraphQL and REST
Both GraphQL and REST facilitate data exchange between client and server in a client-server model, using HTTP as the underlying communication protocol. Here are some similarities:
- Resource-Based Design: Both treat data as resources with unique identifiers. In REST, these are represented by URIs, while in GraphQL, they are defined in the schema and identified by the entities.
- Stateless: Both are stateless architectures, where each request is independent.
- Support for JSON: Both can use JSON for data format, although REST can also support XML and other formats.
Key Differences Between GraphQL and REST
Data Fetching
-
REST: Multiple endpoints can lead to inefficiencies when fetching related data across several resources. For example, fetching a user and their posts might require multiple requests.
-
GraphQL: A single query can retrieve all related data, significantly reducing the need for multiple network requests. This leads to more efficient data fetching and improved performance.
Example: In REST, to fetch a user and their posts, you might need:
GET /users/123
GET /users/123/posts
In GraphQL, you can fetch the same data with a single query:
{
user(id: "123") {
name
email
posts {
title
}
}
}
Performance and Flexibility
- REST: While REST APIs are generally easy to cache and scale, they can suffer from latency issues in complex systems due to multiple round trips. Each endpoint is designed to serve a specific resource, which can lead to inefficient data fetching.
- GraphQL: By reducing the number of requests and allowing for precise data retrieval, GraphQL can offer performance benefits, especially in systems with complex data relationships. It enables clients to specify their exact data needs, reducing the likelihood of redundant data transfers. Code Snippet Example (GraphQL):
{
user(id: "1") {
name
email
posts {
title
comments {
content
author {
name
}
}
}
}
}
Error Handling
- REST: Error handling needs to be implemented by developers. Responses typically include http status codes and error messages, but the structure can vary across different APIs.
- GraphQL: Inbuilt error handling and detailed error messages due to its strong type system. Errors are returned alongside the data, making it easier for clients to understand and handle exceptions.
Versioning
- REST: Often uses versioned endpoints to handle changes, which can be cumbersome.
- GraphQL: No need for versioning; deprecated fields are marked and can be handled gracefully.
When to Use GraphQL vs. REST
Use GraphQL if:
- You need to reduce the number of API calls without creating new REST APIs for different data compositions.
- You have various frontend clients with different data requirements.
- Your application has a rich user interface that changes frequently.
- You want to expose your API to third-party developers for building external applications.
Use REST if:
- You are building simple APIs with well-defined endpoints.
- Your application has low complexity and data interrelations.
- You prefer the simplicity and familiarity of REST.
Summary of Differences: REST vs GraphQL
Aspect | REST | GraphQL |
---|---|---|
Endpoint Structure | Multiple endpoints for different resources | Single endpoint for all operations |
Data Fetching | Fixed data structure, may lead to overfetching or underfetching | Clients specify exact data needed, avoiding overfetching |
Performance | Potentially slower for complex queries due to multiple requests | More efficient for complex queries, reduces multiple requests |
Flexibility | Less flexible, relies on predefined endpoints | High flexibility in querying data |
Data Types | Weakly typed, depends on documentation for consistency | Strongly typed schema, ensures consistency |
Real-Time Data | Requires additional mechanisms for real-time updates | Supports subscriptions for real-time updates |
Caching | Leverages HTTP caching mechanisms (e.g., ETags, cache-control headers) | More complex, requires custom caching strategies |
Versioning | Often requires versioned endpoints to manage changes | No versioning needed, handles changes through schema updates |
Error Handling | Requires custom error handling in the API implementation | Built-in error handling with detailed messages |
Development Speed | Slower for complex queries, requires managing multiple endpoints | Faster development for complex queries and rapid iterations |
Learning Curve | Easier to learn and implement, well-documented and supported | Steeper learning curve due to its flexibility and complexity |
Tooling and Ecosystem | Mature ecosystem with extensive tooling and documentation | Growing ecosystem with strong community support |
Use Cases | Best for simple, well-defined data structures and public APIs | Ideal for complex, interrelated data, and real-time applications |
Scalability | Inherently scalable due to statelessness and simplicity | Requires careful management of queries and resolvers |
GraphQL complements REST
While GraphQL offers an excellent developer experience for frontend developers, it should primarily be used for composing data from multiple sources. REST/RPC APIs remain the best choice for implementing your business logic. Additionally, you should not hand-write your GraphQL layer. Instead, you can use Tailcall to automatically convert your REST APIs to GraphQL.
If you want to know why we think this way, you can read more about it in the article Writing a GraphQL Backend by Hand is Long Gone.
Conclusion
REST is ideal for simple data sources with well-defined resources, using multiple endpoints in the form of URLs. GraphQL excels in handling large, complex, and interrelated data sources with a single URL endpoint, providing flexibility and efficiency.
Choosing between GraphQL and REST depends on the specific needs of your application. While REST offers simplicity and scalability, GraphQL provides unparalleled flexibility and efficiency for complex and dynamic data needs. For many applications, using a hybrid approach that leverages the strengths of both technologies might be the most effective strategy.
Understanding the differences between GraphQL and REST, along with their appropriate use cases, allows you to make informed decisions that optimize both the development process and the end-user experience. By considering the structure of your data, the nature of your client applications, and the specific requirements of your use cases, you can select the API design that best fits your needs.
Explore both GraphQL and REST by implementing them in small projects or integrating them into different parts of a larger system. Experience firsthand how each handles real-world data scenarios to better understand their operational benefits and limitations. This hands-on approach will provide deeper insights into their practical applications and help you make more informed decisions about which technology to adopt for various aspects of your projects.
For quickly creating a GraphQL server that converts REST APIs to GraphQL, check out Getting Started with Tailcall.
By immersing yourself in both the theory and practice of GraphQL and REST, you can develop a robust understanding of how to effectively design and implement APIs that meet the evolving demands of modern applications.